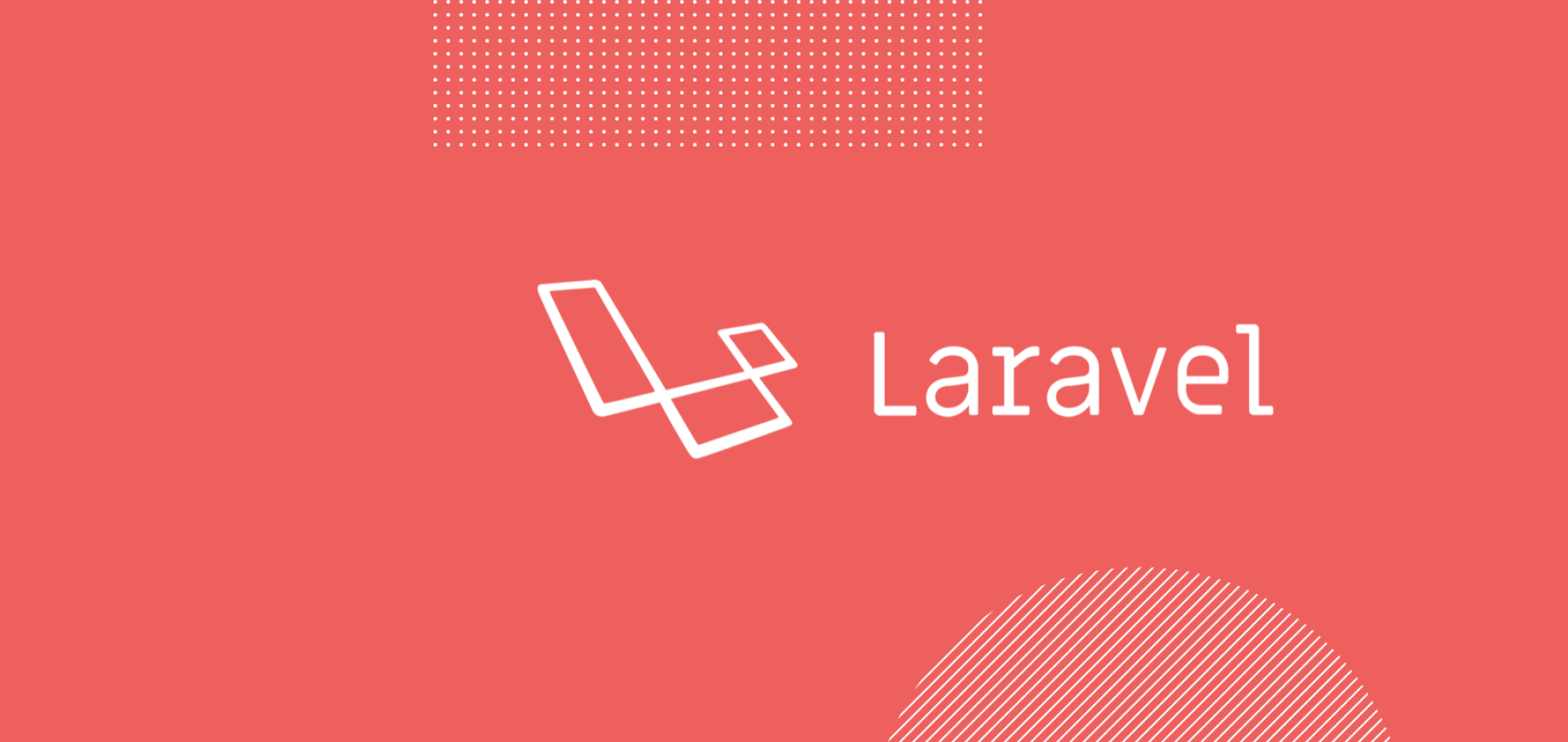
Why is Laravel the best PHP framework?
-
-
Author
Deepak Kundra -
Published
Why Laravel is the Best PHP Framework for SaaS and Cloud Development
In today’s digital-first world, businesses demand robust, scalable, and efficient software solutions. PHP, one of the oldest and most widely used programming languages, continues to power millions of applications across the globe. Among its many frameworks, Laravel has emerged as the standout choice, especially for SaaS and cloud-based application development.
Let’s dive deeper into why Laravel is the preferred framework for modern development needs and how it addresses the challenges faced by SaaS and cloud developers.
1. MVC Architecture for Scalable Applications
Laravel’s Model-View-Controller (MVC) architecture is a cornerstone for building scalable and maintainable SaaS applications. It separates the application logic, UI, and data layers, ensuring a clean codebase that is easier to manage as your application grows.
- Model: Efficiently manage your database interactions and business logic.
- View: Create dynamic, responsive user interfaces.
- Controller: Bridge the gap between the model and view for seamless application behavior.
Example of MVC Implementation in Laravel:
// Model
class User extends Model {
protected $fillable = ['name', 'email', 'password'];
}
// Controller
class UserController extends Controller {
public function index() {
$users = User::all();
return view('users.index', compact('users'));
}
}
// View (resources/views/users/index.blade.php)
@foreach ($users as $user)
<p>{{ $user->name }}</p>
@endforeach
This modularity is essential for cloud-based SaaS applications, where scalability and rapid development cycles are critical.
Docker Compose Configuration for Laravel and MySQL
Using Docker Compose, you can set up a development environment for Laravel with MySQL easily. Here’s an example configuration:
docker-compose.yml
:
version: '3.8'
services:
app:
image: php:8.1-fpm
container_name: laravel_app
volumes:
- ./src:/var/www/html
working_dir: /var/www/html
networks:
- laravel_network
depends_on:
- db
ports:
- "8000:8000"
command: bash -c "composer install && php artisan serve --host=0.0.0.0 --port=8000"
db:
image: mysql:8.0
container_name: mysql_db
restart: always
environment:
MYSQL_ROOT_PASSWORD: root
MYSQL_DATABASE: laravel
MYSQL_USER: laravel_user
MYSQL_PASSWORD: secret
ports:
- "3306:3306"
volumes:
- db_data:/var/lib/mysql
networks:
- laravel_network
volumes:
db_data:
networks:
laravel_network:
.env
File Configuration:
DB_CONNECTION=mysql
DB_HOST=db
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=laravel_user
DB_PASSWORD=secret
Commands to Get Started:
- Build and start containers:
docker-compose up -d
- Access the Laravel application at
http://localhost:8000
.
This setup ensures a seamless development environment for Laravel applications.
2. Robust Authentication and Authorization
Security is a primary concern for any SaaS platform. Laravel offers built-in authentication and authorization features that simplify the process of securing your application. Key benefits include:
- Out-of-the-box Authentication: Easily implement login, registration, password reset, and more.
- Role-Based Access Control (RBAC): Assign specific roles and permissions to users for granular control.
- Enhanced Security: Features like hashed passwords and protection against common vulnerabilities such as SQL injection and XSS.
Authentication Setup Example:
// Enable Authentication Routes
php artisan make:auth
// Controller Example
public function login(Request $request) {
$credentials = $request->only('email', 'password');
if (Auth::attempt($credentials)) {
return redirect()->intended('dashboard');
}
return back()->withErrors(['email' => 'Invalid credentials provided.']);
}
These capabilities ensure your SaaS platform is secure, reliable, and compliant with industry standards.
3. Seamless Integration with APIs and Microservices
Laravel’s flexibility makes it a top choice for integrating with third-party APIs and building microservices-based architectures. Its elegant HTTP client, Laravel HTTP, allows developers to:
- Consume external APIs effortlessly.
- Build RESTful APIs with standardized conventions.
- Enable real-time data exchange using WebSocket or Laravel Echo.
Example of API Integration:
use Illuminate\Support\Facades\Http;
$response = Http::get('https://api.example.com/data');
if ($response->successful()) {
$data = $response->json();
return view('data.index', compact('data'));
}
For SaaS applications that rely heavily on integrations—like payment gateways, CRMs, and analytics tools—Laravel provides the tools and conventions to simplify these tasks.
Conclusion
Laravel is more than just a PHP framework; it’s a comprehensive toolkit for building modern, scalable, and secure SaaS and cloud-based applications. Its rich feature set, coupled with a developer-friendly ecosystem, ensures faster development cycles and robust application performance.
At Duskbyte, we specialize in leveraging Laravel to craft high-performance SaaS solutions tailored to your business needs. Whether you’re building a new platform or modernizing an existing application, Laravel provides the foundation for success in today’s competitive landscape.
Ready to Build Your Next SaaS Solution?
Contact us today to explore how we can bring your vision to life with Laravel.